computer science
An Introduction to Programming

ELEMENTS OF PROGRAMMING (INPUT, OUTPUT, PROCESSING)
In the process of writing a computer program (source code), there are some vital elements that needs to be considered including input (what is coming in ), processing (what operations are you carrying out), output (what results are you expecting). using a diagram,you can see how these three (3) elements relate to each other in figure below.
The popular saying, garbage-in-garbage-out, is actually used to express the idea that in computing (especially when writing programs) and other fields, incorrect or poor-quality input will definitely, produce faulty output.
These 3 elements (mentioned above), form the underlying basis for computing and computers.
Computer input and the input unit
What is Input Devices
An input device (In computing), is a piece of computer hardware equipment used to provide control signals and data to an information processing system such as a computer or information appliance. Examples of input devices include:
-
keyboards,
-
mouse,
-
scanners,
-
digital cameras and joysticks.
Though we will not be covering how input devices work in this section, it is worthy to note that like program translators, which translate high level languages to machine readable forms, input devices also translate keystrokes into signals for the computer to understand using switches and circuits.
What is computer Input
The information you supply to a computer or program (source code) through the input unit or input device. Simply put, input means feeding some data into a program, in the form of a file or from the command line. Most programming languages provide a set of built-in functions to read a given input and feed it to the program as required.
Input in C --integer
#include <stdio.h>
int main() {
int testInteger;
printf("Enter an integer: ");
scanf("%d",&testInteger); ---->input section
printf("Number = %d",testInteger);
return 0;
}
Computer output and the output unit
What is an output Device
An output device (In computing), is An output device is any device that is used to send data one computer to another or to a user. Most data output(from computing devices) meant for humans are in the form of audio, video or text. Examples of output devices include monitors, projectors, speakers, headphones, printers, 3D Printer, Braille embosser, Braille reader, Flat panel, GPS, Headphones and Computer Output Microfilm (COM).
similar to input devices, the output unit has the responsibility of translate signals from the computer to human understandable forms.
What is computer Output
Output is information that has been processed by and sent out from by a computer or program. A common example is what you are viewing on your computer monitor now. Anything that comes out of the computer appearing in a variety of forms -- as binary numbers, as characters, as pictures, and as printed pages can be considered as an output. Generally, any data generated by a computer is referred to as output.
Output in C
#include <stdio.h>
int main() {
int testInteger;
printf("Enter an integer: ");
scanf("%d",&testInteger); ---->input section
printf("Number = %d",testInteger); ---->output section
return 0;
}
Computer Processing and the processing unit
What is a processor
A computer processor or microprocessor, is a small chip ( hardware device) that dwells in computers and other electronic devices. Its major task is to receive input and provide the appropriate output. The CPU (central processing unit)of a computer, is a logic circuitry that responds to and processes all the basic instructions that drive a computer. It handles basic system instructions, such as running application programs, delivery output and processing keyboard/mouse input. Majority of desktop computers come with a CPU (which is responsible for carrying out all tasks). The CPU collaborates with computer programs (software) to execute user instructions (normally coming from the software). Mobile devices ( laptops, phones and tablets), may use processors such as Intel and AMD. Notwithstanding, sometimes these devices use specific mobile processors like
BRAND, Qualcomm, Samsung, Huawei, CHIPSET, Snapdragon 845, Exynos 9810, HiSilicon Kirin 970, Dual-ISP, all of which are designed using the ARM (Advanced RISC Machines) architecture.
The computer works in a parts as shown in the diagram above. The CPU converts data input to information output. through a highly complex, extensive set of electronic circuitry that executes stored program instructions. The CPU consists of two parts: The control unit (which contains circuitry that uses electrical signals to direct the computer system to carry out stored program instructions. The control unit does not execute program instructions; instead, it directs other parts of the system to do so. It must communicate with both the arithmetic/logic unit (ALU) and memory. ) and the arithmetic/logic unit (which contains the circuitry that executes all arithmetic and logical operations such as comparing of numbers). The data storage unit (divided into primary and secondary units) seen in the above diagram, interacts closely with the CPU, for both instructions and data. The computer's (primary) memory holds data temporarily, while the computer is executing a program. but the secondary storage holds permanent or semi-permanent data on some external magnetic or optical medium e.g CD-ROM disks and flash drives.
elements of programming
#include <stdio.h>
int main() {
char chr;
printf("Enter a character: ");
scanf("%c",&chr); ---->input section
printf("You entered %c.",chr);
return 0;
}
Input in C -- Character
number1 = input("Enter a number: ") ---->input section
number2 = input("Enter a second number: ") ---->input section
sum = number1 + number2
print('The sum of {} and {} = {}.format (number1 ,number2))
Input in python -- integer
Input in python -- string
surname= input("Please enter your surname: ") ---->input section
name = input("Now enter you name: ") ---->input section
print("\n Your full name is: \n Surname:\t {}\n Name:\t {}".format(surname, name))
Output in python
surname= input("Please enter your surname: ") ---->input section
name = input("Now enter you name: ") ---->input section
print("\n Your full name is: \n Surname:\t {}\n Name:\t {}".format(surname, name))---->output section
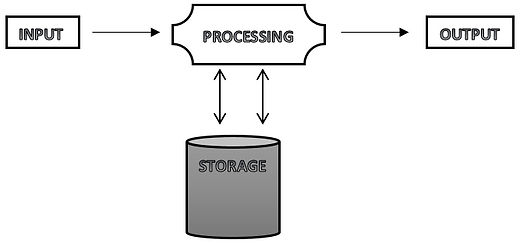