Map, Filter, reduce in Javascript
.map(), .reduce(), and .filter(). are javascripts methods.
These methods are useful, as javascript employs functional programming paradigm.
Functional programming is a style of building the structure and elements of computer programs—that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data.
Functional programming depends heavily on the use of lists and list operations. When a list/array is presented to a Javascript program for instance, the program uses map, filter and reduce functionalities to transform the input array/list to a new form, while keeping the same original list intact.
.map()
Given an array of multiple objects – each object representing an item.
let old_array=[20, 24, 56, 88]
assuming we want to multiply all the items in array
There are multiple ways to achieve this.
1. Using .forEach():
var new_array= [];
old_array.forEach(function (x) { new_array.push(x*x); });
console.log(new_array);
2. using .for():
for(var a of old_array){
new_array.push(a*a);
}
Notice how you have to create an empty array beforehand in the cases above?
Now you see how the program can be simplified to one line.
3. using .map():
new_array= old_array.map(function (x) { return x*x });
console.log(new_array);
We can also make the code simpler by applying the arrow functions
new_array= old_array.map(x => x*x);
console.log(new_array);
Basically .map function takes 2 arguments, a callback and an optional value
The callback runs for each value in the array and returns each new value in the resulting array. The resulting array is always the same length as the original array.
.reduce()
Like the map() function, .reduce() also runs a callback for each element of an array. The different is that reduce() passes the result of this callback to (the accumulator----integer, string, object, etc-----which must be instantiated or passed when calling .reduce()) from one array element to the other.
using our initial array, old_array=[20, 24, 56, 88] for example
We need to know the total of all the item in old_array.
With .reduce(), it’s pretty straightforward:
new_array= old_array.reduce(function (sum, x) { return sum + x; }, 0);
console.log(new_array)
The value 0, is the starting value.
We can also make the code simpler by applying the arrow functions
At the end the reduce function the code will return the final value of the accumulator (which is 188 for this example).
Let’s see how this can be shortened with ES6’s arrow functions:
new_array = old_array.reduce((sum, x) =>sum + x, 0);
Take note, using .reduce() is an easy way to generate a single value or object from an array.
.filter()
The filter function removes unwanted elements from an array/list and return the removed if assigned to a value or the remainder if not.
Using our initial array with additional words or letters, old_array=['a', 20, 'man', 24, 56, 'welcome', 88]
Lets try and return a new array without numbers using .filter()
new_array =old_array.filter(function (val) { return typeof val != 'number'; });
Again, we can use the arrow function for this;
new_array = old_array.filter( val=> typeof val != 'number' );
Alternatively, we can create a seperate funtion for filtering and then pass a callback to the actual filter function
Example:
var old_array=['a', 20, 'man', 24, 56, 'welcome', 88]
function notNumber(val) {
return typeof val != 'number'
}
function state_Function() {
var new_arr=old_array.filter(notNumber);
console.log(new_arr)
document.getElementById("demo").innerHTML =new_arr;
}
state_Function()
FURTHER EXAMPLE:
Exercise:
Return only the workers with salary greater than 1000 pounds from the array below:
var employee = [ { id: 10, name: "Paul Dameon", salary: 800, }, { id: 20, name: "Temmo Wex", salary: 2000, }, { id: 41, name: "Kalim Lintra", salary: 5000, }, { id: 99, name: "Akpova Asty", salary: 600, }, { id: 66, name: "Pahun Linto", salary: 5000, }, { id: 72, name: "Cynthia Ross", salary: 8000, }];
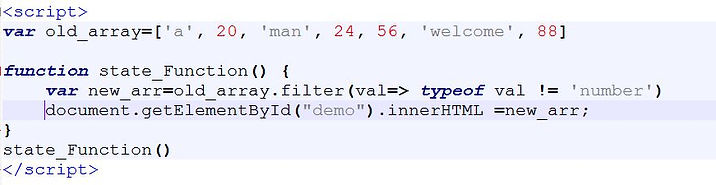
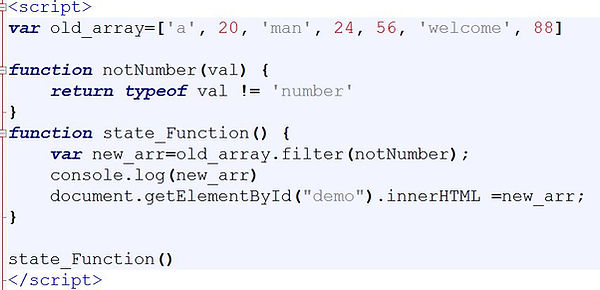
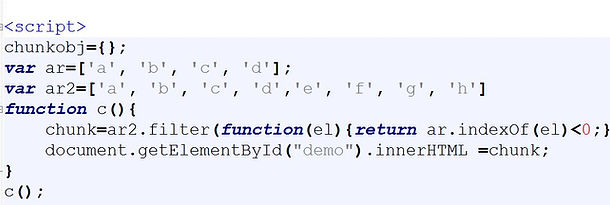